Sudoku#
This problem is taken from PuLP case studies.
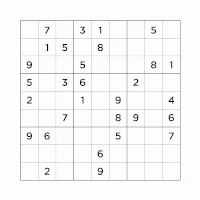
This example shows how we can use flow.Set
with operators flow.set_add
, flow.set_eq
to manipulate set opeartions in OptFlow.
# Author: Anonymized for paper review
# Case Study: Sudoku
# https://coin-or.github.io/pulp/CaseStudies/a_sudoku_problem.html
import optflow as flow
x = flow.Variable(cat=flow.categorical, size=9, shape=(9, 9))
row = [flow.Set(size=9) for _ in range(9)]
col = [flow.Set(size=9) for _ in range(9)]
square = [[flow.Set(size=9) for _ in range(3)] for __ in range(3)]
constraints = []
full_set = flow.Set(size=9, value=range(9))
for i in range(9):
for j in range(9):
row[i] = flow.set_add(row[i], x[i, j])
col[j] = flow.set_add(col[j], x[i, j])
square[i // 3][j // 3] = flow.set_add(square[i // 3][j // 3], x[i, j])
for i in range(9):
constraints.append(flow.set_eq(row[i], full_set))
constraints.append(flow.set_eq(col[i], full_set))
constraints.append(flow.set_eq(square[i // 3][i % 3], full_set))
prob = flow.Problem(constraints=constraints)
prob.solve(solver=flow.metaheuristics)
for i in range(9):
for j in range(9):
print(x[i, j].optimized_value + 1, end=' ')
print()
An example result is as follows
Time elapsed: 2.23 s
2 1 9 5 6 8 4 3 7
4 8 6 7 3 9 5 2 1
3 7 5 4 2 1 6 9 8
7 9 2 3 1 4 8 6 5
8 4 3 6 5 7 2 1 9
5 6 1 9 8 2 7 4 3
1 2 7 8 9 6 3 5 4
9 5 8 2 4 3 1 7 6
6 3 4 1 7 5 9 8 2
[Run Online Demo] (password: )