Welcome to OptFlow 0.1.3 Alpha#
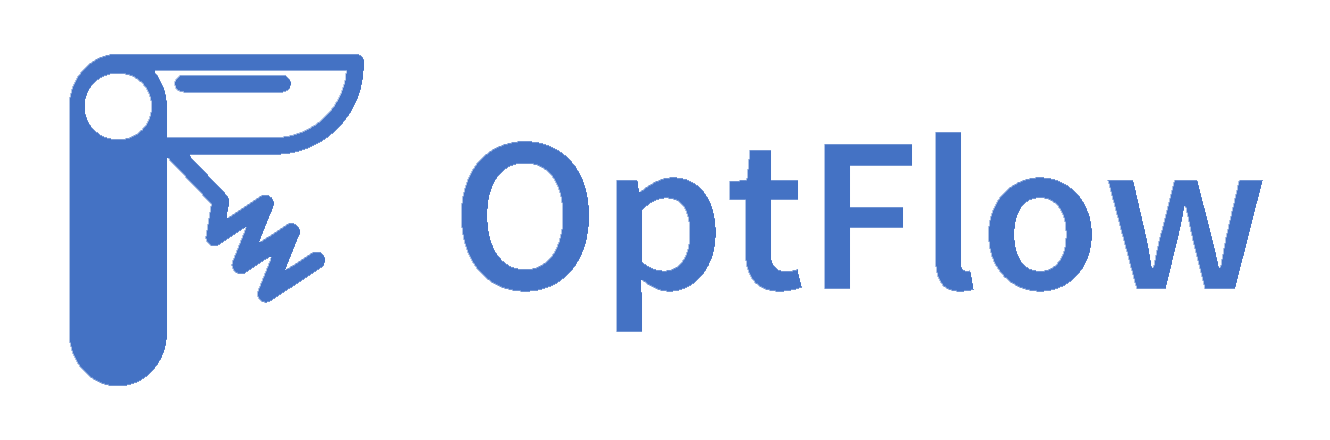
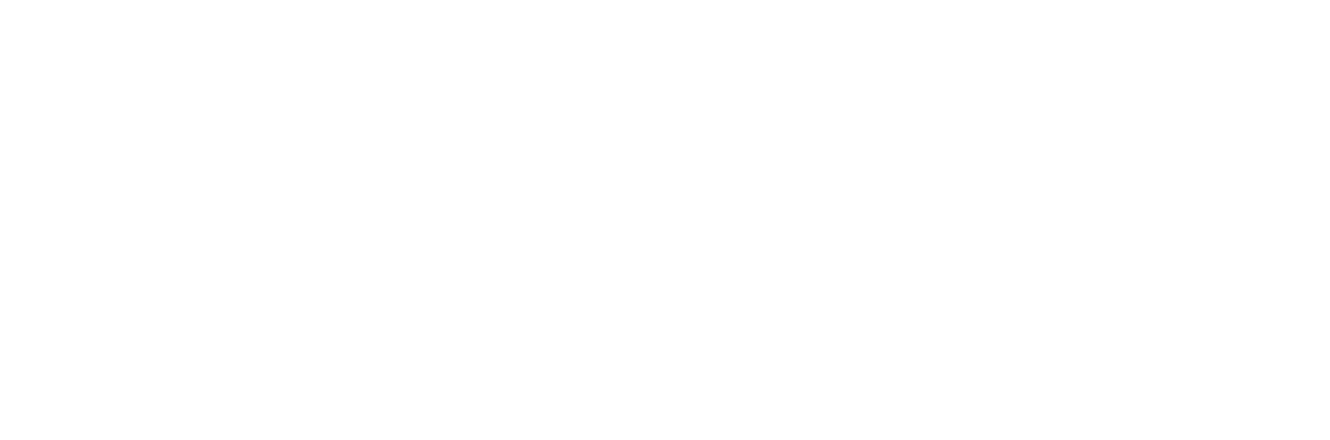
Unified Optimization with Dataflow Graphs
OptFlow is an unified optimization framework integrating multiple optimization methodologies, aiming to solve an unprecedented range of optimization problems in an efficient way. It lets you express your problem in a generic, Turing-complete dataflow graph language, rather than in the restrictive standard form required by different optimization methodologies. You can provide (almost) any computable objective that you want to maximize or minimize, including but not limited to
Optimize the actions of a player to maximize the score of a game (let’s say, CartPole) |
Optimize the selection of items to maximize the total value of a knapsack without exceeding its capacity |
Optimize the number in the blank box to deliver a feasible solution of 9x9 Soduku |
Optimizes the transition function of a Turing machine to maximize the sum of the value on its tape |
OptFlow supports auto-modeling that analyzes the characteristics of your dataflow-graph represented problem, and translates it to the following backend solving methodologies:
… (more methodologies in development)
OptFlow also supports auto-solving that automatically accelerates the solving of your problem by matching it with suitable acceleration techniques, including:
Just-in-time Compilation (JIT) that compile your problem to native machine bytecode to achieve extreme efficiency.
Learning to Optimize (L2O) for problems with specific structures.
In this way, OptFlow let you unlock the power of various optimization methodologies as a whole, and enjoy performance boost seamlessly.
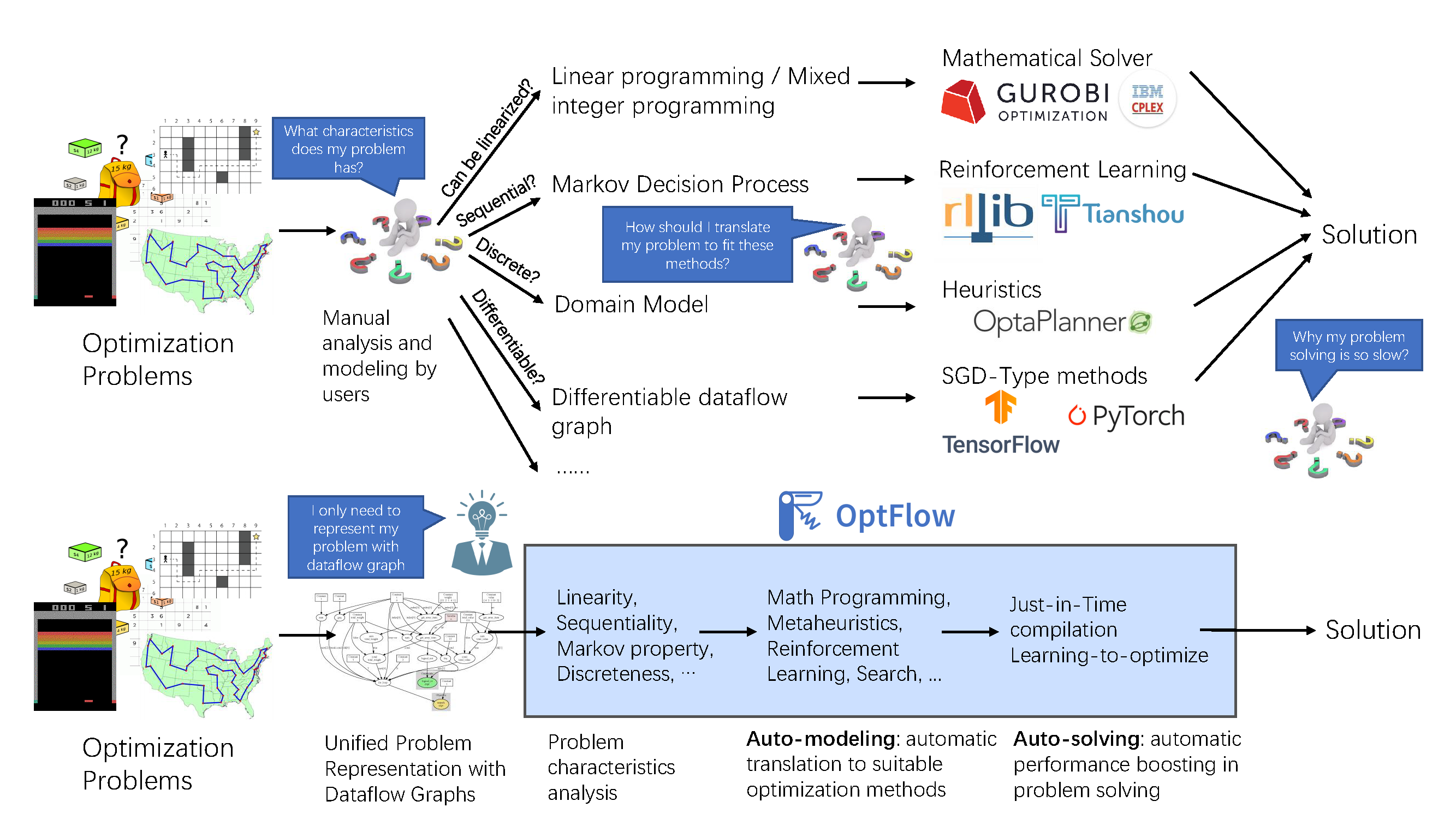
OptFlow pipeline compared with the traditional one.#
Examples#
The following code shows how OptFlow solves the CartPole problem simulated by Gym
import optflow as flow
from optflow.contrib.gym_op import Gym, GymStep, GymReset
gym_init = Gym('CartPole-v1')
gym_reset = GymReset()
gym_step = GymStep()
# Construct the problem with dataflow graph
env = gym_init()
env, state = gym_reset(env)
total_reward = flow.Constant(0.)
x = flow.Variable(cat=flow.categorical, size=2, shape=(flow.infinite, )) # actions to be optimized
with flow.ForLoop(flow.infinite) as t: # create a loop operation to run the game
env, state, reward, done = gym_step(env, x[t]) # play one step of the game
total_reward += reward # accumulate the reward
with flow.IfCond(done): # create a condition operation to check if the game is terminated
flow.break_loop() # break the game loop
prob = flow.Problem(objective=total_reward, sense=flow.maximize)
# Train a model as reinforcement learning method is selected
prob.train(trainer=flow.rl, params={'num_episodes': 100})
# The optimal objective value is returned by `prob.solve()`.
opt_obj = prob.solve(solver=flow.rl)
# The optimal value for x is stored in `x.optimized_value`.
print(x.optimized_value)
[Run Online Demo] (password: )
and the following code shows how OptFlow solves the knapsack problem with multiple optimization methodologies
import optflow as flow
import numpy as np
# Problem data
N, max_weight = 5, 10
weight = flow.Constant(value=np.array([2, 2, 6, 5, 4]))
value = flow.Constant(value=np.array([6, 3, 5, 4, 6]))
# Construct the problem with dataflow graph
x = flow.Variable(cat=flow.binary, size=N, shape=(N, ))
obj = flow.sum([value[i] * x[i] for i in range(N)])
constraints = [flow.sum([weight[i] * x[i] for i in range(N)]) <= max_weight]
prob = flow.Problem(objective=obj, constraints=constraints, sense=flow.maximize)
# Solve the problem with multiple optimization methodologies
opt_obj_prog = prob.solve(solver=flow.programming) # solve via mathematical programming
opt_obj_mh = prob.solve(solver=flow.metaheuristics) # solve via metaheuristics
opt_obj_search = prob.solve(solver=flow.search) # solve via search
print(opt_obj_prog, opt_obj_search, opt_obj_mh, x.optimized_value)
[Run Online Demo] (password: )
While both the two short scripts construct the problem with dataflow graph, they involve four optimization methodologies: reinforcement learning, mathematical programming, metaheuristics and search.
More examples can be found in the Examples page.
Table of Contents#
- Install
- User Guide
- Basic of OptFlow Dataflow Graph
- Describing Optimization Problems using Dataflow Graph
- Solving Optimization Problems with Different Optimization Methodologies
- Describing Sequential Optimization Problems using Dataflow Graph
- Solving Sequential Optimization Problems with DP and RL
- JIT Compilation to Accelerate Solving
- Technical Details
- Examples
- API Documentation
- Subpackages
- Submodules
- optflow.analyzer module
- optflow.constants module
- optflow.executor module
- optflow.mdp module
- optflow.operators module
BaseNode
Constant
ConstantSlice
CustomOperator
ForLoop
Graph
GraphAgent
IfCond
Input
InputSlice
NodeBundle
Operation
Set
Variable
VariableSlice
abs()
add()
break_loop()
cond()
debug_print()
eq()
for_loop()
geq()
graph_agent_location()
graph_agent_move_to()
graph_distance()
gt()
identity()
leq()
logical_and()
logical_not()
logical_or()
lt()
maximum()
minimum()
mul()
ne()
neg()
rand()
reduce_sum()
set_add()
set_eq()
set_in()
set_not_in()
sub()
sum()
unpack()
watch_variable_name()
- optflow.problem module
- Module contents
- Citing OptFlow